Hi,
Im having trouble to read data from port 30002 and 30004, I am already able to use port 29999 and send petitions and read data. Not sure if its done in a different way.
Following I have my client code in C# to connect to 29999 port and by changing its location to 30004/2 it does connect but the commands I send don't return the values specified.
CODE:
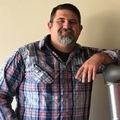
You have to unpack the stream, can’t just convert to a string. The docs on the UR website show you how each packet of the stream has been packed, it’s different depending on the message type. Also, port 30004 is the RTDE port, you have to actually send the setup to the port before it will stream anything back to you. There are good docs on the support site with a python example that we used to base our rtde client on.
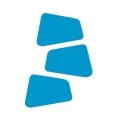
Thanks Matthew for your time. Ill try with port 30002 (seems easier), do you know of any open source code for the unpacking of the output stream from the robot?matthewd92 said:You have to unpack the stream, can’t just convert to a string. The docs on the UR website show you how each packet of the stream has been packed, it’s different depending on the message type. Also, port 30004 is the RTDE port, you have to actually send the setup to the port before it will stream anything back to you. There are good docs on the support site with a python example that we used to base our rtde client on.
Thks again.
Hi,