There is a way to do that, you open a TCP connection to port 30003 and can then send the command. Ill post up here when I get back to my laptop the function that we created to set the speed slider. We just call it at the top of the program to set it wherever we want, so if I'm debugging I may set it slow and then I can speed up the robot manually. If I have a long program but there's a particular spot I want to go slow I can automatically slow it down so I can see what's happening in a small section for instance.
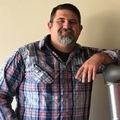
@johan92lee it is and it isn't, it's special in that it is not a URScript command that can just run as part of a program but it's not special in that it is basically just the command that is sent to the controller when you move the speed slide, here is more information. I have picked up these little tidbits just reading through the UR support site over several years and gaining a really good understanding of what is happening behind the scenes with the robot and the touch pendant. Another thing that we use are the dashboard commands, both within the program and as remote tools. For instance, if I want to have a customer test a new program we will load it onto the robot's memory remotely and then we can use a dashboard command to not only load the program but then tell the program to play for instance. This is all done across port 29999 and there is a list of commands that are available to use here. Another example where we use the dashboard server is to have popup messages that are non-blocking and only display an OK button, so purely informational. An example is, on long loading programs where there is a lot happening in the Before Start portion of the program such as an intricate homing routine we might pop up a message saying something like, "Please wait while the program prepares to start" so that the operator knows that something is happening and doesn't keep pressing the play button. We will then at the end of the Before Start dismiss the popup so to ensure that it is not still there.
My best recommendation for learning all the great little things that can be done is to read through all of the FAQ's and How-To's on the UR Support site which can be found at https://www.universal-robots.com/support/
I will start a thread where maybe everyone can post up any little functions that they have written that help simplifies using the robot such as the runSlow function above.
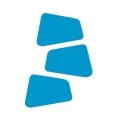
Got it. Thanks for your patience.
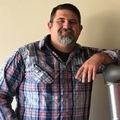
No worries, glad you got it working. I’ve never understood why they hide that like they do.
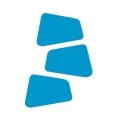
matthewd92
Do you know of any way to lock in the speed slider when the robot is being moved manually from the move screen? My goal would be to only allow operators to move the robot at a reduced speed.
When the UR is in run mode, does the program speed slider default to 100%? Also, is there a way to use a script function of some sort to verify or set the speed slider to 100%? I am using the UR in a sealant dispensing application where the UR picks the part and moves it underneath a stationary needle. We had one cycle where it dispensed too much sealant because the UR was going slower than normal but I believe the UR was running in the program tab and the speed slider may have been lowered by accident.