Make a simple program with gripper and release command. Than look at the .script file generated after executing the program. You will see in the script how SET and GET functions are used.
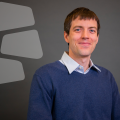
Just mention the original article about how to control gripper connected on UR robot using socket communication.
https://dof.robotiq.com/discussion/2420/control-robotiq-gripper-mounted-on-ur-robot-via-socket-communication-python#latest
Here below is the definition of ePIck URscript functions which can be found in the .script file generated when you execute a program.
By looking at the definition of those function you will be able to understand which GET and SET command you can send to control ePick.
Look at rq_set_var and rq_get_var functions.
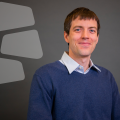
I never tested to control ePick this way but ePick and 2F or hande are controlled using similar commands.
Here below are the registers for ePick and 2F:
As you can see it is almost the same. Registers are just used differently.
- The "Position request" register of the 2F become "Max relative pressure level request" for ePick.
- The "Speed" register of the 2F become "Grip timeout/release delay" for ePick.
- The "Force" register of the 2F become "Minimum relative pressure level request" for ePick.
Here below is a compairison of action request register:
- 2F
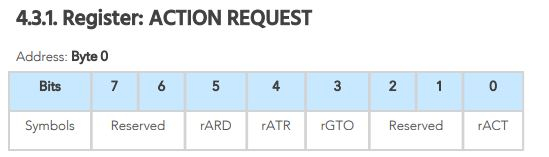
- ePick
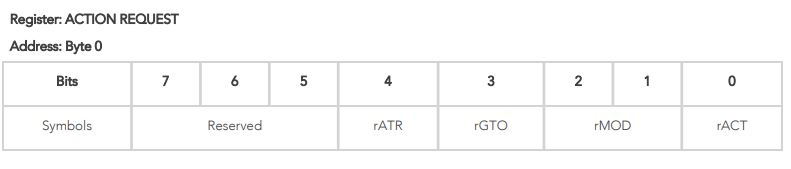
The main difference is that ePick action request register bit 1and 2 are use to set the vacuum mode "rMOD".
Looking at this, if would pass the following commands to actuate ePick:
#activate ePick
SET ACT 1
#Set go to bit to 0. This reset the gripper action request.
SET GTO 0
#Set gripper mode. 0 for Automatic mode OR 1 for Advanced mode. In this example we set advanced mode to manually set minimum and maximum vacuum. If you use Automatic mode no need to set min an max vacuum level.
SET MOD 1
#Set max vacuum level. 0 for 0%. 255 for 100%.
SET POS 255
#Set min vacuum level. 0 for 0%. 255 for 100%.
SET FOR 70
#Set go to bit to 1. The gripper will execute the action.
SET GTO 1
ePick manual will help you to understand the details:
https://assets.robotiq.com/website-assets/support_documents/document/EPick_Instruction_Manual_e-Series_PDF_20210709.pdf
I have robotiq 2f-85 and epick. I followed https://dof.robotiq.com/discussion/2489/problem-on-control-robotiq-gripper-mounted-on-ur-robot-via-socket-communication-python and ur-rtde. And I am able to control the 2f-85 on UR5 and UR10 properly, getting feedback and position accurately. But for the 'epick' it's not working properly. I am not able to understand what command should be SET and GET for the can be used for Epick, Like 'SET POS 0-255' is for 2f-85 and should not be working with Epick. With activate, both grippers get activated. Can you explain the proper use of the GET and SET commands so that I can turn it ON, OFF and get the object is present or not.