Fantastic, this is exactly what I was looking for! I have had many issues while connecting through python and this works.
I can perform almost everything! Thank you so much!
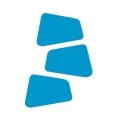
Hi @bcastets, this looks fantastic. Does this method works on the Robotiq E-pick vaccum gripper?
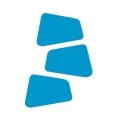
Hi @bcastets I installed two grippers onto UR5e via socket connection using the adaptor plate. My questions is, does port 63352 point to both grippers? If yes, how can I write my code to control them separately? If not, what is the port for the other gripper?
Thanks.
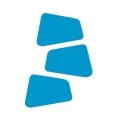
Hi @bcastets, this looks fantastic. Does this method works on the Robotiq E-pick vaccum gripper?
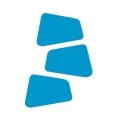
hi
@bcastets I got this from this code.
Gripper finger position is: b'POS ?\n'.
it seems like some format error
Do you know why is that?
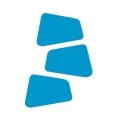
@@bcastetsbcastets said:@aumax
Could you precise how you are sending your code ?
just follow the code above. what I used was ur3e and 2f-85 gripper and set ur to remote control
#Library importation
import socket
#Socket setings
HOST="192.168.12.101" #my ur ip
PORT=63352 #PORT used by robotiq gripper
#Socket communication
with socket.socket(socket.AF_INET, socket.SOCK_STREAM) as s:
#open the socket
s.connect((HOST, PORT))
s.sendall(b'GET POS\n')
data = s.recv(2**10)
#Print finger position
#Gripper finger position is between 0 (Full open) and 255 (Full close)
#Gripper finger position is between 0 (Full open) and 255 (Full close)
print('Gripper finger position is: ', data)
and I got
Gripper finger position is: b'POS ?\n'.
and my question is: the ? should be something else, right? like 1 2 or something if it works correctly
and I got
Gripper finger position is: b'POS ?\n'.
and my question is: the ? should be something else, right? like 1 2 or something if it works correctly
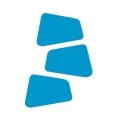
hi
@bcastets I got this from this code.
Gripper finger position is: b'POS ?\n'. it seems like some format errors
Do you know why is that?
aumax said:hi
@bcastets I got this from this code.
Gripper finger position is: b'POS ?\n'. it seems like some format errors
Do you know why is that?
@bcastets Thank you for super easy approach! Works like a charm.
Has been a long time since the post went up but better late than never. Anyone facing problem where the output is something like this b'POS ?\n', make sure to check if the gripper ID is set to 1. In case it is not, either change it to 1 or additional steps would be required to send the commands along with the gripper ID.
I would like to share with you some python code which can be use to control a gripper connected to a UR robot from an external PC using python and a socket connection.
The gripper URCAP works with a server running on the robot. The server receive the messages send by the program and manage the modbus RTU communication with the gripper.
It is possible to send message to the gripper URCAP server directly from a PC using a socket connection.
Here below is an example of python code to get the finger position:
#############################################################
#Gripper finger position is between 0 (Full open) and 255 (Full close)
As you can see it is quite simple. Just need to send "GET POS" to the URCAP server to get the gripper position.
The server can receive GET and SET requests.
Example of GET request:
GET POS
Example of SET request:
SET POS 100
Here below are some possible commands. Some commands can be use with both GET and SET:
Similar post:
https://dof.robotiq.com/discussion/2291/send-and-get-data-from-ur-robot-and-control-robotiq-grippers-via-rtde-using-python-3-ur-rtde#latest