Here is a video showing a UR robot controlled with this program:
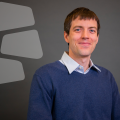
This is a problem with your joystick and the integration with pygame Joystick (https://www.pygame.org/docs/ref/joystick.html).
Looking at the error message it looks like your joystick does not have axis 3.
I would recommend that you start making a simple python program trying to get and display axis position. This way you can identify the axis of your joystick and adjust my program is needed.
The program should run under 3.5, some little adjustment maybe required.
It is a side comment but after this project, I learned that using speedL script command in the UR program would be better to have a fluid motion. With my program the robot progress step by step.
I would like to share with you a little program I made to control UR robot using a joystick connected on a PC.
Here is how it works:
A python program is running on my pc. It catchs the position of joystick axis and write this position in UR robot modbus server input register.
On the robot, a program is running. It calculates the next position of the robot depending on the position of the joystick and order the next move.
Python script and ur program are available on github:
https://github.com/castetsb/urJoystickControl