I am not very familiar with what is capable to be done using the palletizing wizard (we don't really use it, depend a lot on scripts for stuff like this) but I can see a way to do this in script and make a relatively easy program but a few questions first. Are all 4 part numbers stacked the same pattern, just different box sizes? If not, how much do the patterns vary?
We have done similar type applications, not palletizing, but packaging where we had to make determinations on orientation based on some pattern. You can use the modulo operator to help with that determination and then use some simple math to make the pattern.
So assuming that we want to stack the first layer, we would want to know the box height, width and length. Let's call this layer 0 and lets start with column 0, so the bottom pink row.
I am going to assume that we have a feature with the origin at the front left corner of the pallet (corner of the pink box in the middle of the image) and that X runs to my left, Y runs to the back and then Z runs up. This will be the coordinate system that we will use to determine where to place each box.
So here is some psuedo code and how I would think about doing the task
EDIT: I tried to format this as a code snippet but it would not show the whole thing so here it is as plain text
##############################################################
# Some constants that I would ask the operator at the start of the program
width (X direction)
length (Y direction)
height (Z Direction)
totalBoxes Total number of boxes per layer
numberOfcolumns Number of columns per layer
numberBoxesInLongColumn (pink columns)
numberBoxesInShortColumn (yellow columns)
originOfPallet (stored as a feature)
numberOfLayers
#Some variables that we will need to keep track of where we are in the process, I would make these installation variables
columnCount = 0
rowCount = 0
layerCount = 0
# First lets determine which layer we are on, even or odd so we know where the pink row goes
if (layer%2 == 0):
layerName = "even"
elif( layer%2 == 1):
layerName = "odd"
end
#Pick box from conveyor
Some move here to get box from the conveyor to near the box
#Start doing math to determine how to place box
if (layerName == even):
if (columnCount == 0):
xDistance = width
yDistance = length
zRotation = 1.57 (90 degrees in radians)
longRow = True
else:
xDistance = length
yDistance = width
zRotation = 0
longRow = False
end
else:
if (columnCount == 3):
xDistance = width
yDistance = length
zRotation = 1.57 (90 degrees in radians)
longRow = True
else:
xDistance = length
yDistance = width
zRotation = 0
longRow = False
end
end
xLocation = 0.5*xDistance + xDistance*columnCount
yLocation = 0.5*yDistance + yDistance*rowCount
zLocation = layerCount*height
boxLocation = pose_trans(originOfPallet,p[xLocation, yLocation, zLocation, 0, 0, zRotation])
overBoxLocation = pose_trans(boxLocation, p[0,0,height,0,0,0])
#Use variable waypoint and move to the box location
moveJ(overBoxLocation)
moveL(boxLocation)
set vacuum off
moveL(overBoxLocation)
# Move back over conveyor
#Keep track of all the counters
rowCount = rowCount + 1
if (longRow):
if rowCount > numberBoxesInLongColumn:
rowCount = 0
columnCount = columnCount +1
end
else:
if rowCount > numberBoxesInShortColumn
rowCount = 0
columnCount = columnCount +1
end
end
if columnCount > numberOfColumns:
layerCount = layerCount + 1
columnCount = 0
if layerCount > numberOfLayers:
layerCount = 0
popup("Please remove the completed pallet and place a new empty pallet, THEN press CONTINUE")
end
end
##############################################################
This is a very quick example based on the single image above but hopefully you can get the idea of how you could write a rather simple program to provide the pallet stacking like you are showing in the image above.
If you venture this and try it, let us know how it works out please.
Hey all, we are looking at modifying a current UR10 program that is picking up boxes from a conveyor and stacking them on 2 skids. There are 4 different box sizes with different ways of being stacked on the skids. Currently they are using a make-shift palletizing program with long scripts defining fixed points for each drop position. My question is whether or not there is a simple way of utilizing the palletizing wizard to orientate the boxes shown in the image below. I have searched online but find nothing for a cross stacked pallet as such. I really don't want to have to create 5 different 2D pallet programs for each skid as that will add up fast for each different box.
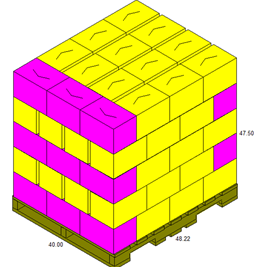
Any input is appreciated, thank you.