Are you trying to use the Polyscope circle move? If so, you need two circle moves to make a 360 degree circle, 3 is even better, that is because you cannot do more than 180 degrees with the circle move. Its best to limit it to 120 degrees. We use them with pretty good success, you can choose the locked scenario when you are setting up the circle move and that will lock the torch orientation to be the starting torch orientation. The trick is keeping that same orientation for each of the circle moves.
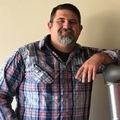
Actually you do not need a start point for the second arc, you use a point to get to the arc (inside the movep) and then the movec only takes 2 points, the via point and the end point, then you place the second arc segment with a via point and a end point.Zach said:I have been using the polyscope circle move. Would you suggest using the endpoint of the first arc as the start of the second arc, or use the script command get_actual_tcp_pose() after the endpoint of the first move as the start of the second arc?
Here is an explanation from UR that does a pretty good job. https://www.universal-robots.com/how-tos-and-faqs/how-to/ur-how-tos/circle-using-movec/
You will see that point 5 is the same as point 1 so they are only breaking the circle into 2 halves.
MoveP
waypoint1
moveC
waypoint2 #first via point (90 degrees)
waypoint3 #Midppoint of full circle (180 degrees)
moveC
waypoint4 # second via point (270 degrees)
waypoint1 # end waypoint also the same as the start point
All,
I've been really struggling how to do a 360 degree circle move with my tcp fixed to the orientation from the first point. Has anyone had success with this? If so how?