I think the simplest solution is to use the robot modbus registers.
From your raspberry pi you can read or write information in robot modbus registers.
You can easily find modbus communication library on the web. This can be done in Python for example.
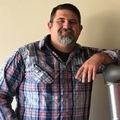
Another option is to use socket communications, socket servers are pretty easy to create in most languages, you could even use SocketTest as a simple server to test with. You can find a download of that program here
To make this work you could do something like concat the variable name with the value in that variable and then send through the socket to the server where it would know how to decompile the value received into the name and value
def sendVariableToSocket(name, value):
local valueToSend = str_cat(str_cat(name,":"), value)
socket_open("xxx.xxx.xxx.xxx", portNumber, "temporary")
socket_send_string(valueToSend, "temporary")
socket_send_byte(10, "temporary")
socket_close("temporary")
end
To use the function do something like
local myVariable=3
sendVariableToSocket("myVariable", myVariable)
This would send a string to the socket server something like "myVariable:3" at which point you could split the string into the value and the name by splitting on the ":" delimiter.
I have an application where I would like to be able to send things like program names or variables from the robot to a arduino/ raspberry pi/ laptop. What would be the simplest way to send that data from the robot?