If the force is zero it is normal to have 0amp current. You need to have more than 0 force to have current continuously feed to the motor. Current reading cannot be use to do things like gripping force estimation.
I recommand you play with our PC interface to understand how works the gripper. In this interface it is posible to see the modbus registers of the gripper.
https://assets.robotiq.com/website-assets/support_documents/document/RUI-2.4.6_20210412.zip
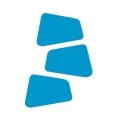
Thank you for your quick answer. I have now noticed that the COU register is only assigned a value while the gripper is moving and it is set back to zero once the gripper reaches its target position or it detects an object. But, what then is the exact meaning of this register? Surely the gripper draws more current when it is holding an object and thus exerting force upon that object, than when the gripper is simply open, though COU reads zero in both cases as long as the gripper has stopped moving. Consider also section 4.3.6 of the 2F-85 & 2F-140 - Instruction Manual:bcastets said:If the force is zero it is normal to have 0amp current. You need to have more than 0 force to have current continuously feed to the motor. Current reading cannot be use to do things like gripping force estimation.
I recommand you play with our PC interface to understand how works the gripper. In this interface it is posible to see the modbus registers of the gripper.
https://assets.robotiq.com/website-assets/support_documents/document/RUI-2.4.6_20210412.zip
"The force setting defines the final gripping force for the gripper. The force will fix the maximum current sent to the motor. If the current limit is exceeded, the fingers stop and trigger an object detection notification. Please refer to the Picking Features section for details on force control."
It would seem then that internally the gripper current is indeed used for gripping force estimation, as force limits are translated to current limits? Is this gripper current not the same one made accessible in the COU register?
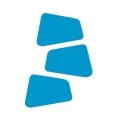
Thank you for your quick answer. I have now noticed that the COU register is only assigned a value while the gripper is moving and it is set back to zero once the gripper reaches its target position or it detects an object. But, what then is the exact meaning of this register? Surely the gripper draws more current when it is holding an object and thus exerting force upon that object, than when the gripper is simply open, though COU reads zero in both cases as long as the gripper has stopped moving. Consider also section 4.3.6 of the 2F-85 & 2F-140 - Instruction Manual:bcastets said:If the force is zero it is normal to have 0amp current. You need to have more than 0 force to have current continuously feed to the motor. Current reading cannot be use to do things like gripping force estimation.
I recommand you play with our PC interface to understand how works the gripper. In this interface it is posible to see the modbus registers of the gripper.
https://assets.robotiq.com/website-assets/support_documents/document/RUI-2.4.6_20210412.zip
"The force setting defines the final gripping force for the gripper. The force will fix the maximum current sent to the motor. If the current limit is exceeded, the fingers stop and trigger an object detection notification. Please refer to the Picking Features section for details on force control."
It would seem then that internally the gripper current is indeed used for gripping force estimation, as force limits are translated to current limits? Is this gripper current not the same one made accessible in the COU register?
Hello there, I have a Robotiq 2F-85 attached to a UR3e robot, the robot is connected to my laptop via a wired ethernet network. I'm using python code very similar to a different post to control the gripper over a socket connection, link and minimal code example below. I can set target position, speed, force etc without issue, I can read back the actual position, gripper status etc, BUT: when I try to read the motor current, it always returns zero, regardless of whether or not the gripper is holding something. Any idea what might be the problem? Is there some action that must be taken for the motor current to be written to its respective register?
Code source:
https://dof.robotiq.com/discussion/2420/control-robotiq-gripper-mounted-on-ur-robot-via-socket-communication-python
Code:
robotiq2f_tcp.py
main.py
output
COU 0
This output is also obtained when the robot is first made to grasp an object (using functions not included in the above code example).